Demystifying Concurrency, Parallelism, and Asynchronous Programming in .NET: Understanding the Key Differences
Your complete guide to discovering the nuances of concurrency, parallelism, and async in .NET. Learn key differences & see code examples to enhance performance & responsiveness in your applications.
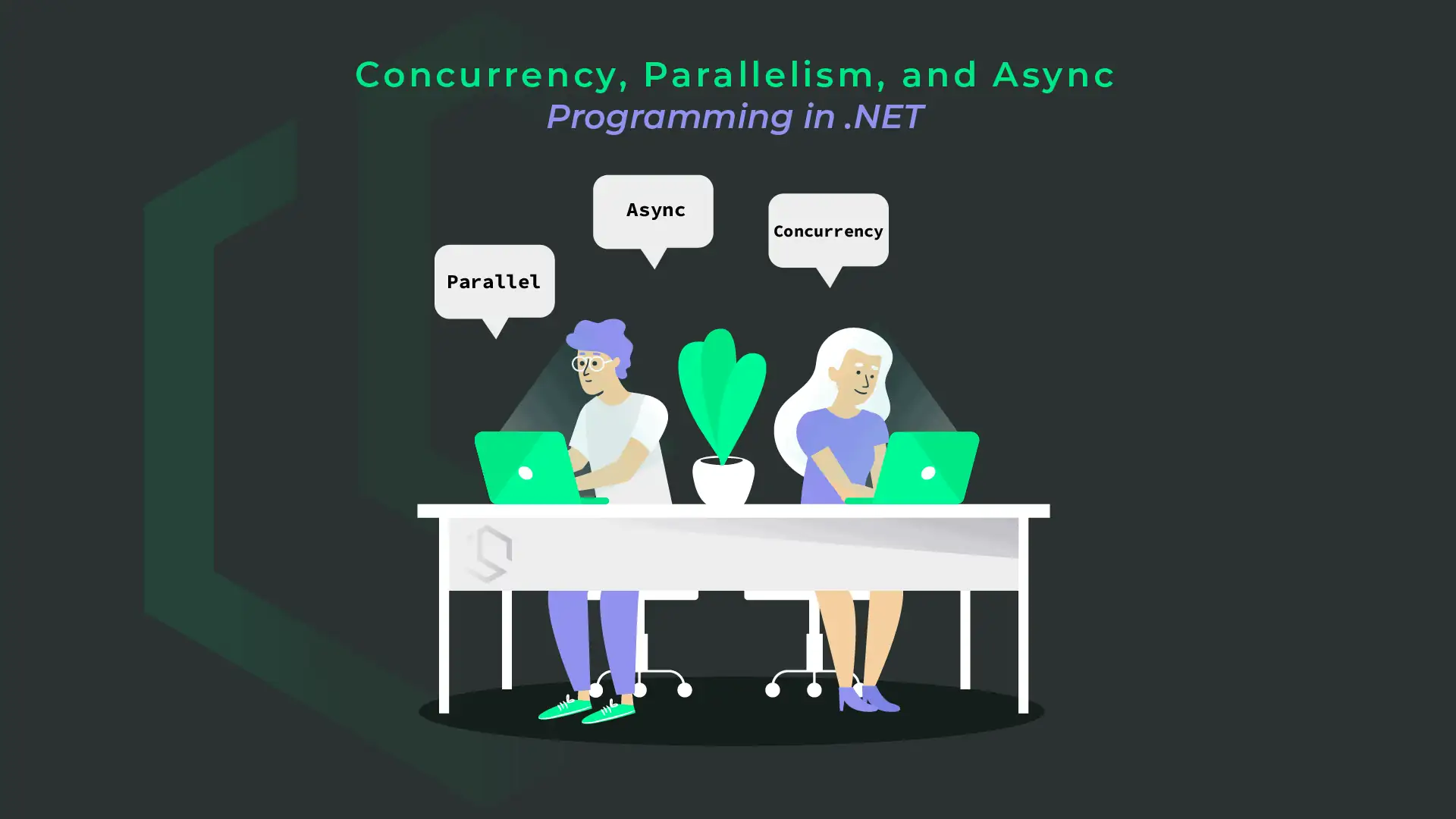
I have often seen the question, what is the difference between concurrency, parallelism, and asynchronous programming? I totally get that there's confusion when just thinking of each of the three. In this blog post, I will provide you with a clear understanding of the differences between concurrency, parallelism, and asynchronous programming in .NET, and help you choose the right approach for your .NET applications based on their specific requirements.
Introduction
Concurrency, parallelism, and asynchronous programming are often used interchangeably, but they have distinct differences when it comes to .NET development. Understanding these differences is crucial for writing efficient and effective .NET applications. In this blog post, we will delve into the definitions of concurrency, parallelism, and asynchronous programming in the context of .NET, and explore their benefits, challenges, and common use cases.
Definition of Concurrency💡
Concurrency refers to the ability of a system to handle multiple tasks simultaneously, without necessarily executing them simultaneously. In the context of .NET, concurrency involves managing multiple threads or tasks that can be performed concurrently, but not necessarily in parallel. Concurrency allows for overlapping of tasks, where multiple tasks can be in progress at the same time, but not necessarily executing at the same time.
Definition of Parallelism💡
Parallelism, however, refers to the simultaneous execution of multiple tasks using multiple threads or processors. In parallelism, tasks are divided into smaller subtasks that can be executed independently and concurrently on separate threads or processors, leading to potential speedup and improved performance.
Definition of Asynchronous💡
Programming Asynchronous programming, commonly used with the async
and await
keywords in C#, enables the execution of tasks concurrently without blocking the calling thread. It allows a single thread to handle multiple tasks concurrently by using asynchronous I/O operations or asynchronous programming patterns. Asynchronous programming allows for efficient resource utilization and improved scalability, as threads can be freed up to perform other tasks while waiting for I/O operations to complete.
Importance of Understanding the Differences
Understanding the distinctions between concurrency, parallelism, and asynchronous programming in .NET is crucial for writing efficient and scalable applications. Choosing the right approach for a particular scenario can greatly impact the performance and responsiveness of the application. Incorrect usage of these concepts can lead to issues such as race conditions, deadlocks, and poor performance, which can be challenging to diagnose and fix. Trust me! 😅
In the next sections, I will explore each of these concepts in more detail, providing you with examples of their implementation in .NET, and discussing their benefits, challenges, and common use cases. With a clear understanding of concurrency, parallelism, and asynchronous programming in .NET, you will be equipped to make informed decisions when designing and implementing concurrent and parallel processing in your .NET applications. Let's dive in! 🙌
Concurrency
Concurrency in .NET involves managing multiple tasks or threads that can be executed concurrently, allowing for the overlapping of tasks without necessarily executing them simultaneously.

Here are some examples of concurrent programming techniques in .NET.
Using Task.Run for Concurrent Execution
The Task.Run
method in .NET allows you to start a new task on a separate thread, which can be executed concurrently with the calling thread. Here's an example:
using System;
using System.Threading.Tasks;
public class ConcurrencyExample
{
public static async Task Main()
{
Console.WriteLine("Main Thread: Start");
// Start a new task using Task.Run
Task task1 = Task.Run(() => PrintNumbers());
// Continue executing other tasks concurrently
Console.WriteLine("Main Thread: Continuing");
// Wait for the task to complete
await task1;
Console.WriteLine("Main Thread: End");
}
public static void PrintNumbers()
{
for (int i = 1; i <= 5; i++)
{
Console.WriteLine($"Task Thread: Number {i}");
Task.Delay(1000).Wait(); // Simulating some work
}
}
}
In the example above, the PrintNumbers
method is executed concurrently on a separate thread using Task.Run
, allowing the main thread to continue executing other tasks concurrently. The output may show interleaved lines from the main thread and the task thread, indicating concurrent execution. 💪
Using async and await for Concurrency
Asynchronous programming with async and await keywords in C# allows for concurrency without blocking the calling thread, making it efficient for I/O-bound operations. Here's an example:
using System;
using System.Net.Http;
using System.Threading.Tasks;
public class ConcurrencyExample
{
public static async Task Main()
{
Console.WriteLine("Main Thread: Start");
// Start multiple tasks concurrently
Task task1 = DownloadAsync("https://example.com/file1");
Task task2 = DownloadAsync("https://example.com/file2");
// Continue executing other tasks concurrently
Console.WriteLine("Main Thread: Continuing");
// Wait for all tasks to complete
await Task.WhenAll(task1, task2);
Console.WriteLine("Main Thread: End");
}
public static async Task DownloadAsync(string url)
{
using (var httpClient = new HttpClient())
{
var response = await httpClient.GetAsync(url);
var content = await response.Content.ReadAsStringAsync();
Console.WriteLine($"Downloaded from {url} with content length {content.Length}");
}
}
}
In the example above, DownloadAsync
method is executed concurrently using async
and await
, allowing the main thread to continue executing other tasks concurrently without blocking. The Task.WhenAll
method is used to wait for all tasks to complete.
Benefits and Challenges of Concurrency
Concurrency in .NET can provide benefits such as improved responsiveness, better resource utilization, and the ability to perform multiple tasks concurrently. However, it also presents challenges such as potential race conditions, deadlocks, and difficulty in debugging and diagnosing issues related to concurrent execution. Proper synchronization techniques, such as using locks, monitors, or other synchronization primitives, are important to prevent such issues.
Common Scenarios Where Concurrency is Useful
Concurrency can be useful in various scenarios in .NET, such as parallel processing of data, handling multiple client requests in a server application, performing background tasks concurrently with the main thread, and improving the performance of I/O-bound operations. It's important to carefully analyze the requirements of the application and choose the appropriate concurrency techniques based on the specific use case.
Parallelism
Parallelism (Not the same as Parallel) in .NET involves dividing a task into smaller sub-tasks that can be executed concurrently on multiple threads, typically for performance optimization.
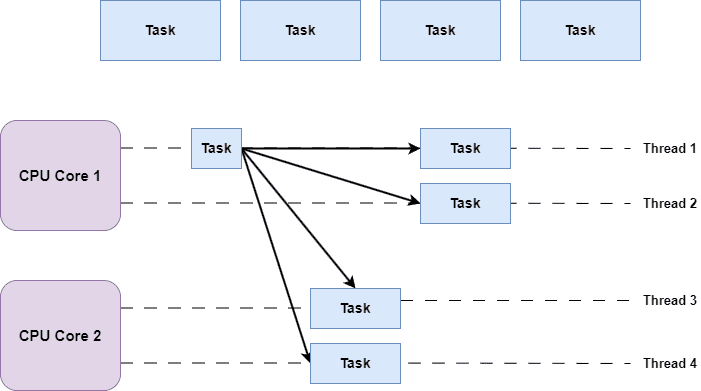
Here are some examples of parallel programming techniques in .NET:
Using Parallel.ForEach for Parallel Execution
The Parallel.ForEach
method in .NET allows you to iterate over a collection and execute a delegate for each element concurrently on multiple threads. Here's an example:
using System;
using System.Threading.Tasks;
public class ParallelismExample
{
public static void Main()
{
Console.WriteLine("Main Thread: Start");
int[] numbers = { 1, 2, 3, 4, 5 };
// Parallel execution using Parallel.ForEach
Parallel.ForEach(numbers, number =>
{
Console.WriteLine($"Task Thread: Number {number}");
Task.Delay(1000).Wait(); // Simulating some work
});
Console.WriteLine("Main Thread: End");
}
}
In the example above, the Parallel.ForEach
method is used to iterate over an array of numbers and execute the delegate concurrently on multiple threads. The output may show interleaved lines from the main thread and the task threads, indicating parallel execution.
Using Parallel.Invoke for Parallel Execution
The Parallel.Invoke
method in .NET allows you to execute multiple delegates concurrently on multiple threads.
Here's an example:
using System;
using System.Threading.Tasks;
public class ParallelismExample
{
public static void Main()
{
Console.WriteLine("Main Thread: Start");
// Parallel execution using Parallel.Invoke
Parallel.Invoke(
() => PrintNumbers(1, 3),
() => PrintNumbers(4, 6),
() => PrintNumbers(7, 9)
);
Console.WriteLine("Main Thread: End");
}
public static void PrintNumbers(int start, int end)
{
for (int i = start; i <= end; i++)
{
Console.WriteLine($"Task Thread: Number {i}");
Task.Delay(1000).Wait(); // Simulating some work
}
}
}
In this example, the Parallel.Invoke
method is used to execute three delegates concurrently on multiple threads, allowing for parallel execution of the PrintNumbers
method.
Benefits and Challenges of Parallelism
Parallelism in .NET can provide benefits such as improved performance, better utilization of multi-core processors, and the ability to process large datasets or perform complex computations in parallel. However, it also presents challenges such as potential race conditions, deadlocks, and difficulty in debugging and diagnosing issues related to parallel execution. Proper synchronization techniques, such as using locks, monitors, or other synchronization primitives, are important to prevent such issues.
Common Scenarios Where Parallelism is Useful
Parallelism can be useful in various scenarios in .NET, such as processing large datasets, performing complex computations that can be divided into smaller tasks, and optimizing performance in CPU-bound operations. It's important to carefully analyze the requirements of the application and choose the appropriate parallelism techniques based on the specific use case.
Asynchronous Programming
Asynchronous programming in .NET allows you to write code that can perform tasks concurrently without blocking the main thread, resulting in improved scalability and responsiveness in applications.
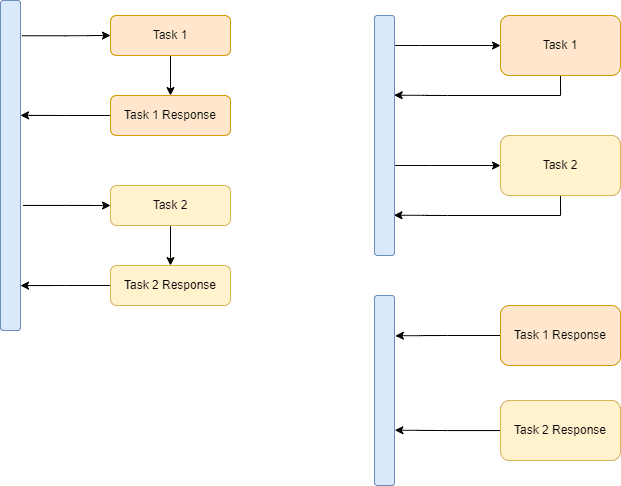
Here are some examples of asynchronous programming techniques in .NET:
Using async/await for Asynchronous Execution
The async
and await
keywords in C# provide a convenient way to write asynchronous code that can execute tasks concurrently without blocking the main thread. Here's an example:
using System;
using System.Net.Http;
using System.Threading.Tasks;
public class AsynchronousProgrammingExample
{
public static async Task Main()
{
Console.WriteLine("Main Thread: Start");
// Asynchronous execution using async/await
await DownloadWebContentAsync();
Console.WriteLine("Main Thread: End");
}
public static async Task DownloadWebContentAsync()
{
using (var client = new HttpClient())
{
string result = await client.GetStringAsync("https://example.com");
Console.WriteLine($"Task Thread: Downloaded content of length {result.Length}");
}
}
}
In the example above, the DownloadWebContentAsync
method uses the await
keyword to asynchronously download web content without blocking the main thread, allowing for concurrent execution of other tasks.
Using Task.Run for Offloading CPU-bound Work
The Task.Run
method in .NET allows you to offload CPU-bound work to a separate thread, freeing up the main thread for other tasks. Here's an example:
using System;
using System.Threading.Tasks;
public class AsynchronousProgrammingExample
{
public static async Task Main()
{
Console.WriteLine("Main Thread: Start");
// Offloading CPU-bound work using Task.Run
await Task.Run(() => CalculateFibonacci(40));
Console.WriteLine("Main Thread: End");
}
public static int CalculateFibonacci(int n)
{
if (n <= 1)
return n;
else
return CalculateFibonacci(n - 1) + CalculateFibonacci(n - 2);
}
}
In this example, the Task.Run
method is used to offload the CPU-bound Fibonacci calculation to a separate thread, allowing the main thread to continue executing other tasks concurrently.
Benefits and Challenges of Asynchronous Programming
Asynchronous programming in .NET can provide benefits such as improved scalability, responsiveness, and resource utilization in applications. It allows for concurrent execution of tasks without blocking the main thread, leading to better performance in scenarios where tasks are I/O-bound or involve waiting for external resources. However, it also presents challenges such as potential issues with exception handling, error handling, and debugging due to the asynchronous nature of the code.
Common Scenarios Where Asynchronous Programming is Useful
Asynchronous programming can be useful in various scenarios in .NET, such as performing I/O-bound operations such as web requests, database queries, and file I/O, and offloading CPU-bound work to separate threads to avoid blocking the main thread. It's important to carefully analyze the requirements of the application and choose the appropriate asynchronous programming techniques based on the specific use case.
In the next section, I will compare and contrast the differences between concurrency, parallelism, and asynchronous programming in .NET, and when to use each approach depending on the requirements of the application.
Key Differences
While concurrency, parallelism, and asynchronous programming may seem similar, they have key differences in how they handle tasks and utilize resources in .NET applications. Understanding these differences can help you choose the appropriate approach for your application's requirements. Let's explore the key differences between these concepts:
Concurrency
Concurrency in .NET refers to the ability of multiple tasks or threads to make progress independently without blocking each other. Concurrency is achieved through techniques such as interleaved execution, where tasks are scheduled and executed in small time slices by the operating system. In concurrent programming, tasks can start and make progress, but not necessarily complete in parallel.
One key difference with concurrency is that it doesn't necessarily mean tasks are executed simultaneously. For example, if multiple tasks are scheduled to run concurrently on a single thread, they will be time-sliced and interleaved, meaning each task takes turns executing without truly running in parallel.
Here's an example of concurrency using async
and await
in .NET:
using System;
using System.Threading.Tasks;
public class ConcurrencyExample
{
public static async Task Main()
{
Console.WriteLine("Main Thread: Start");
// Concurrent execution using async/await
Task task1 = PrintNumbersAsync("Task 1", 10);
Task task2 = PrintNumbersAsync("Task 2", 10);
await Task.WhenAll(task1, task2);
Console.WriteLine("Main Thread: End");
}
public static async Task PrintNumbersAsync(string taskName, int count)
{
for (int i = 1; i <= count; i++)
{
Console.WriteLine($"{taskName}: {i}");
await Task.Delay(100); // Simulate async I/O-bound operation
}
}
}
In the example above, two tasks (task1
and task2
) are executed concurrently using async
and await
, but they are not necessarily running in true parallel. They may be time-sliced and interleaved on the same thread.
Parallelism
Parallelism in .NET refers to the ability of multiple tasks or threads to execute simultaneously on multiple CPU cores. Parallelism is achieved by distributing tasks across multiple threads or processes, allowing them to execute in true parallel and potentially complete faster compared to concurrent execution.
Parallelism is beneficial for CPU-bound tasks that can be divided into smaller, independent subtasks that can be executed simultaneously on multiple CPU cores. It can lead to improved performance and faster completion of tasks, especially in scenarios where tasks involve heavy computational workloads.
Here's an example of parallelism using Parallel.ForEach
in .NET:
using System;
using System.Threading.Tasks;
public class ParallelismExample
{
public static void Main()
{
Console.WriteLine("Main Thread: Start");
// Parallel execution using Parallel.ForEach
int[] numbers = { 1, 2, 3, 4, 5 };
Parallel.ForEach(numbers, num =>
{
Console.WriteLine($"Number: {num}, Thread ID: {Task.CurrentId}");
CalculateSquare(num);
});
Console.WriteLine("Main Thread: End");
}
public static void CalculateSquare(int num)
{
// Simulate CPU-bound workload
for (int i = 0; i < 100000000; i++)
{
num = num * num;
}
Console.WriteLine($"Square: {num}, Thread ID: {Task.CurrentId}");
}
}
In the example above, the Parallel.ForEach
method is used to execute the CalculateSquare
method in parallel for each number in the numbers
array, potentially utilizing multiple CPU cores for faster execution.
Asynchronous Programming
Asynchronous programming in .NET refers to the ability to perform tasks concurrently without blocking the calling thread, allowing for efficient utilization of resources and improved responsiveness in applications. Asynchronous programming is commonly used for I/O-bound tasks, such as reading from or writing to a file or making network requests, where waiting for the task to complete would block the thread and degrade performance.
Asynchronous programming in .NET is typically achieved using the async
and await
keywords, which allow you to write asynchronous code in a more sequential and intuitive manner. Here's an example of asynchronous programming using async
and await
in .NET:
using System;
using System.Net.Http;
using System.Threading.Tasks;
public class AsynchronousProgrammingExample
{
public static async Task Main()
{
Console.WriteLine("Main Thread: Start");
// Asynchronous execution using async/await
HttpClient httpClient = new HttpClient();
string response = await httpClient.GetStringAsync("https://jsonplaceholder.typicode.com/posts/1");
Console.WriteLine($"Response: {response}");
Console.WriteLine("Main Thread: End");
}
}
In this example, the GetStringAsync
method of the HttpClient
class is called asynchronously using await
, allowing the main thread to continue executing other tasks without blocking. Once the response is received, the code after await
is resumed and executed.
What to choose for my application?
Now that we have covered concurrency, parallelism, and asynchronous programming in .NET, let's summarize the key differences between them, to get a better understanding of what to choose for our applications:
- Execution Model - Concurrency allows tasks to start and make progress independently, but not necessarily complete in parallel. Parallelism allows tasks to execute truly in parallel on multiple CPU cores, potentially leading to faster completion. Asynchronous programming allows tasks to be executed concurrently without blocking the calling thread, improving responsiveness.
- Resource Utilization - Concurrency may not utilize multiple CPU cores as tasks may be time-sliced and interleaved on the same thread. Parallelism utilizes multiple CPU cores, leading to better utilization of resources. Asynchronous programming allows the calling thread to continue executing other tasks while waiting for asynchronous operations to complete, avoiding blocking and improving resource utilization.
- Use Cases - Concurrency is suitable for scenarios where tasks can make progress independently and do not require true parallel execution, such as I/O-bound tasks. Parallelism is suitable for CPU-bound tasks that can be divided into smaller, independent subtasks that can be executed in parallel for improved performance. Asynchronous programming is suitable for I/O-bound tasks where blocking the calling thread would degrade performance, allowing for concurrent execution without blocking.
In conclusion, understanding the differences between concurrency, parallelism, and asynchronous programming in .NET is essential for choosing the right approach for your application's requirements. Whether it's I/O-bound tasks that can benefit from concurrency and asynchronous programming, or CPU-bound tasks that can benefit from parallelism, choosing the appropriate approach can greatly impact the performance and responsiveness of your application. 🔥
Summary
In this blog post, I've explored the differences between concurrency, parallelism, and asynchronous programming in .NET. I've discussed their execution models, resource utilization, and use cases, highlighting the key distinctions between them.
Concurrency allows tasks to start and make progress independently, but not necessarily complete in parallel. Parallelism enables tasks to execute truly in parallel on multiple CPU cores, potentially leading to faster completion. Asynchronous programming allows tasks to be executed concurrently without blocking the calling thread, improving responsiveness.
Understanding these differences is crucial for choosing the right approach for your application's requirements. If you have I/O-bound tasks, concurrency, and asynchronous programming may be appropriate, allowing for concurrent execution without blocking the calling thread. For CPU-bound tasks, parallelism can improve performance by utilizing multiple CPU cores.
By leveraging the right approach, you can optimize the performance and responsiveness of your .NET applications. It's important to carefully consider the nature of your tasks and the requirements of your application to determine the most suitable approach for your specific use case.
I hope this blog post has provided you with a clear understanding of the differences between concurrency, parallelism, and asynchronous programming in .NET, and has helped you in choosing the right approach for your future projects. Keep in mind that mastering these concepts and effectively implementing them in your code can greatly enhance the performance and efficiency of your .NET applications. Happy coding! ✌️
Additional Resources
If you're interested in learning more about concurrency, parallelism, and asynchronous programming in .NET, here are some additional resources that you may find helpful:
- Microsoft Documentation: Parallel Programming - This official documentation from Microsoft covers parallel programming in .NET, including topics like Parallel LINQ (`PLINQ`),
Parallel.ForEach
, and more. - Microsoft Documentation: Asynchronous Programming - This official documentation from Microsoft offers a comprehensive guide to asynchronous programming in .NET, including using
async
/`await`,Task
, and other related concepts. - "Concurrency in C# Cookbook" by Stephen Cleary - This book provides practical recipes for implementing concurrency and parallelism in C# and .NET, covering topics like managing threads, synchronization primitives, asynchronous programming, and more.
- "Async in C# 5.0: Unleash the Power of Async" by Alex Davies - This book offers an in-depth exploration of asynchronous programming in C# 5.0 and later versions, covering topics like async/await, Task Parallel Library (TPL), and more.
- "Parallel Programming with Microsoft .NET" by Colin Campbell, Ralph Johnson, and Ade Miller - This book provides a comprehensive overview of parallel programming concepts in .NET, including topics like parallel algorithms, coordination and synchronization, and performance considerations.
These resources can help you further deepen your understanding of concurrency, parallelism, and asynchronous programming in .NET, and provide practical guidance for implementing these concepts in your own code. As with any technology, it's important to continuously learn and stay updated with best practices and new advancements to effectively utilize these powerful techniques in your applications. Happy learning! 🎓