In modern software development, applications are often designed to be configurable, allowing them to adapt to different environments and scenarios without requiring changes to the code itself. 🙌
In .NET Core, configuration data is stored in the IConfiguration
interface, which is used to retrieve key-value pairs from various configuration sources such as appsettings.json
files or environment variables.
Understanding how to retrieve values from IConfiguration
is essential for any .NET Core developer. In this blog post, I will provide a comprehensive guide on how to get values from IConfiguration
in .NET Core, covering the basics of IConfiguration
, the different techniques for retrieving values, and best practices for using IConfiguration
in your applications.
Whether you are a seasoned .NET Core developer or just getting started, this post will provide you with the knowledge you need to work effectively with IConfiguration
and create more flexible and adaptable applications. Let's get started! 🔥
If you are in the market for using the configuration values using IOptions where configurations are loaded into settings classes, then check out my post about how to use IOptions
to bind your configurations.
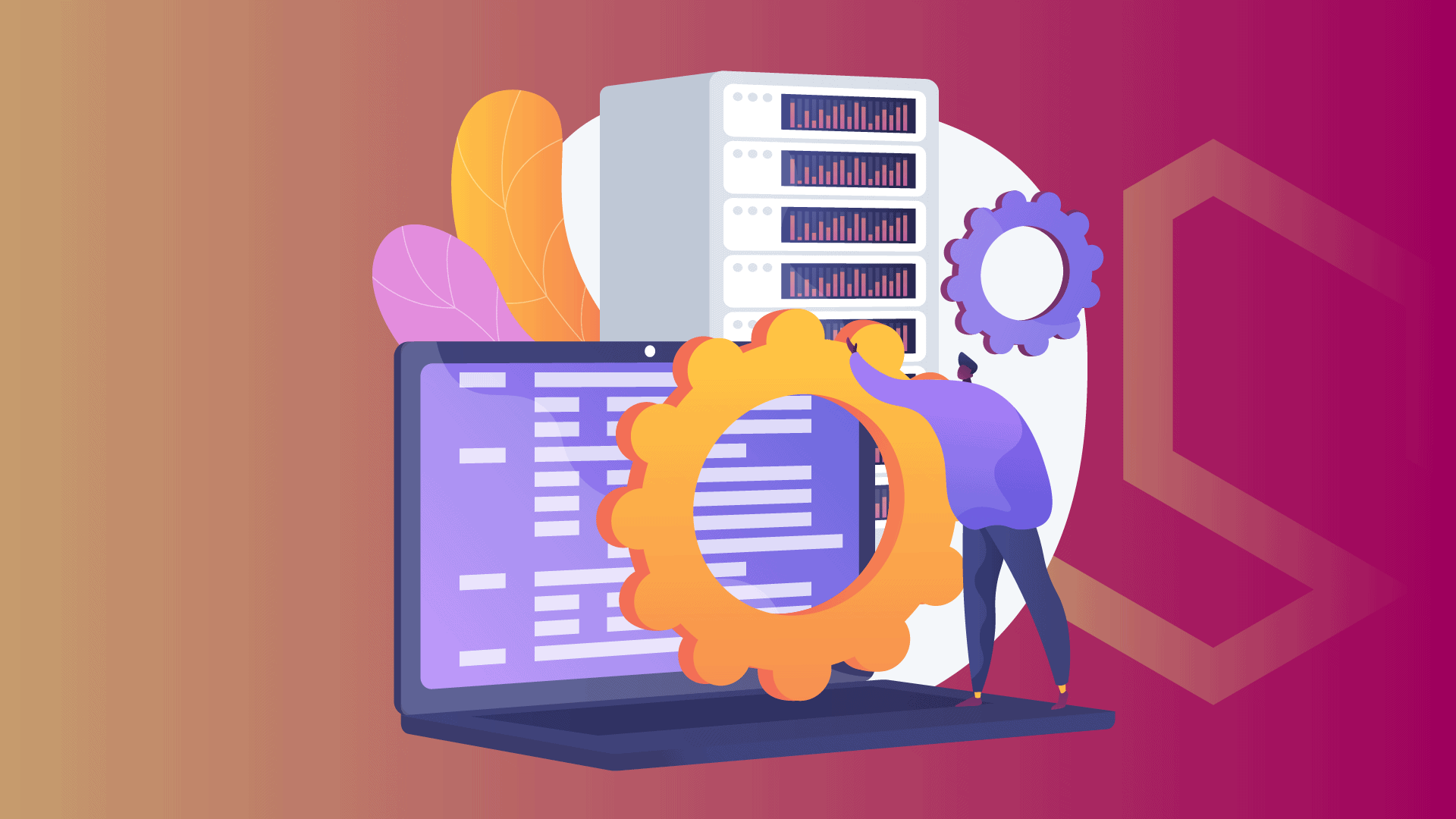
An overview of IConfiguration
Configuration data is a crucial part of any application (I think every developer can agree on this 😅 ), as it allows developers to customize their application's behavior and adapt it to different environments or scenarios. In .NET Core, configuration data is managed through the IConfiguration
interface, which is implemented by various configuration providers.
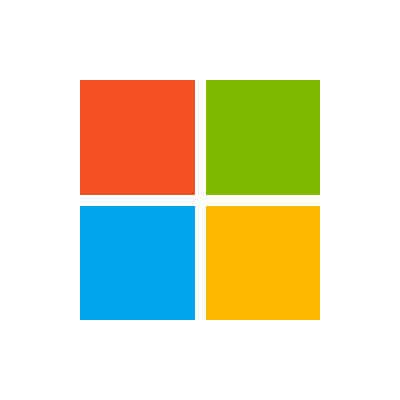
The IConfiguration
interface provides a unified way of accessing configuration data, regardless of the source of the data. This means that developers can retrieve configuration data from different sources such as JSON files, environment variables, or command-line arguments, using the same interface and syntax.
The IConfiguration
interface includes several methods that allow developers to retrieve configuration values based on their key, including GetSection()
, GetValue()
, and Bind()
.
- The
GetSection()
method returns a section of configuration data based on its key, which can then be used to retrieve specific configuration values within that section. - The
GetValue()
method retrieves a configuration value based on its key and can be used for both simple values (such as strings or numbers) and complex types (such as arrays or objects). - The
Bind()
method allows developers to bind configuration values to objects, simplifying the process of reading and parsing configuration data into strongly-typed objects.
In addition to these methods, .NET Core provides various configuration providers, such as JsonConfigurationProvider
, EnvironmentVariablesConfigurationProvider
, and CommandLineConfigurationProvider
, which allow developers to retrieve configuration data from different sources.
By understanding the basics of IConfiguration
and its various configuration providers, you can easily manage your application's configuration data and make your applications more flexible and adaptable to different environments and scenarios. 💪
How to retrieve Values from IConfiguration
Retrieving values from IConfiguration
is a straightforward process, but the technique used depends on the type of data being retrieved and how it's structured in the configuration file.
In this section, I'll explore some of the different techniques for retrieving values, including binding and using indexers, and provide examples of how to retrieve values from IConfiguration
in different scenarios. Let's get our hands dirty! 🧑💻
Retrieving a Simple Value
To retrieve a simple value, such as a string
or an int
(number), you can use the GetValue()
method, as shown in the following example.
string connectionString = Configuration.GetValue<string>("ConnectionStrings:DefaultConnection");
In this code example, we retrieve the value of the DefaultConnection
key from the ConnectionStrings
section of the configuration file.
Retrieving a Complex Type
To retrieve a complex type, such as an array
or an object
, you can use the GetSection()
method to retrieve the section that contains the data and then use the Get<T>()
method to convert the section to the desired type, as shown in the following example.