What is the difference between a POCO, DTO, Entity, and a VO?
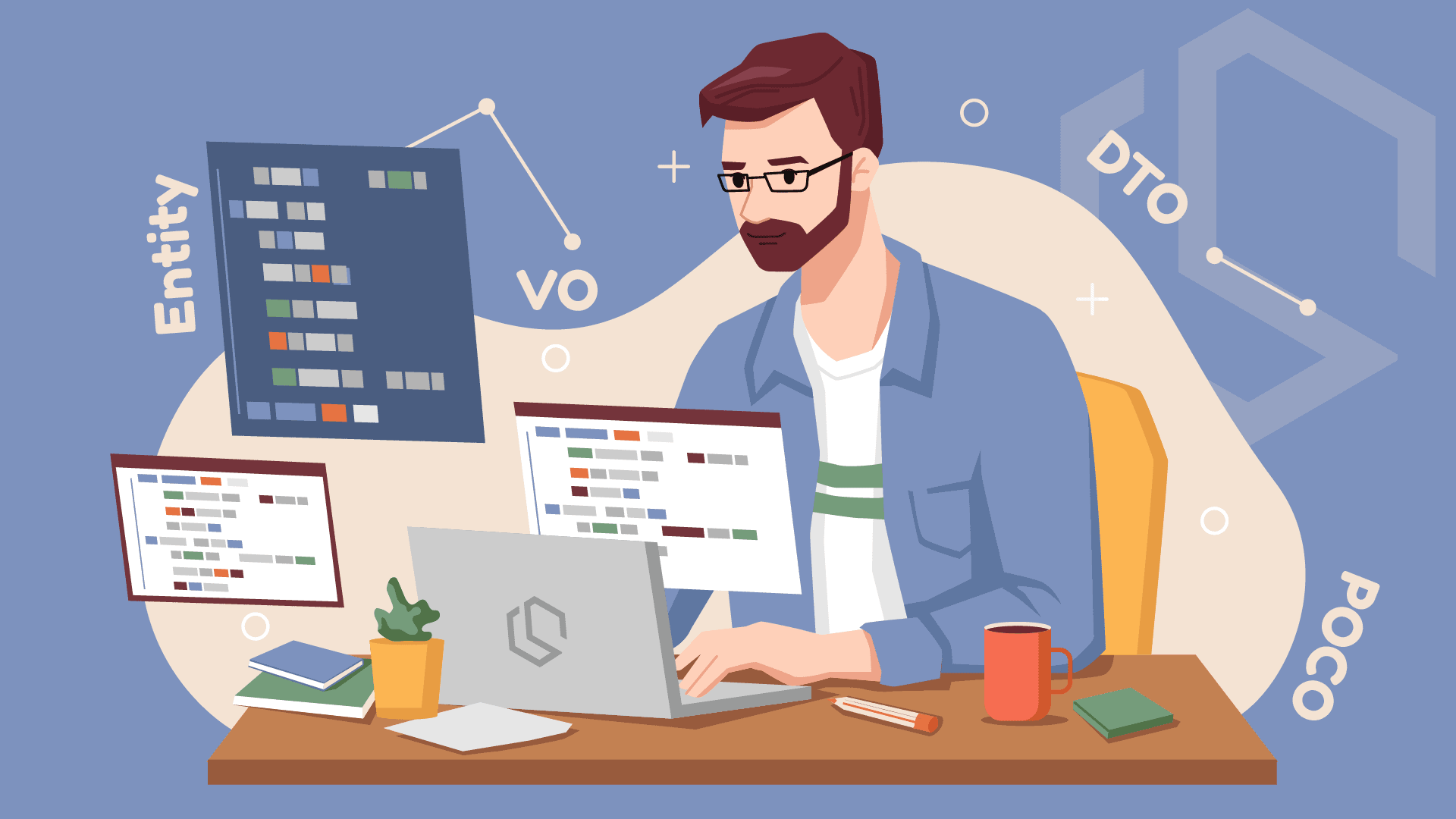
A lot of developers are asking themselves this question. What is the difference between a POCO, DTO, Entity, and a VO? One thing they have in common is that they are classes.
Classes are one of the most basic concepts when doing software development. They define our models/domain for the application and can help us do an infinite number of things. When talking about data traveling around in our software solutions, we got a lot of names and conventions, and they can be confusing for beginners - I totally get that! What the heck do all these terms mean? 🤷
By the end of this post, you will have a good understanding of what the different names mean and how they are used during software development. If you are ready, let's begin! 🤩
Classes And Their Responsibilities
Each type of class got a different purpose. Below is a brief description of each class and its implementation. It's important to say that this can change from language to language, but often it will be the same for the most popular programming languages.
POCO - Plain Old CLR Objects
Let's start by having a look at a POCO class to understand what we are dealing with.
public class Customer
{
public virtual int Id { get; set; }
public virtual string Name { get; set; }
public virtual string Address { get; set; }
public virtual ICollection<Order> Orders { get; set; }
}
A Plain Old CLR Object (POCO) is a class, which doesn't depend on any framework-specific base class. They are somewhat in a family with an Entity, but the core difference is that a POCO does not have any behavior.
By saying that a POCO doesn't have any behavior I mean that it implements only the domain business logic of the Application. These could be models for database tables, etc... They will contain no validation, rules, and so on.
Entity
An entity is semantic - it is relational to something with meaning in a language or logic. An entity is something that exists virtually or physically. An entity can be something like:
- Teacher (Can be both physical and virtual)
- Book (Can be virtual)
- Product (Can be virtual)
- Patient (Can be both physical and virtual)
- Car (Physical)
- Video (Virtual)
- Customer (Can be both physical and virtual)
I think you get the point. Entities are mutable objects that encapsulate business logic. When using entities in C# we often talk about code first - this means that we write our entities and their relations using code in the application and then create the database based on those entities. In other words - they are 100% related to tables in a database or a domain unit virtually or physically.
When making repositories in an application we often work with entities because they are responsible for the operations in the database, from 3rd-party data sources, etc... Below is an example of a set of entities with relations.
public class Customer
{
public string Name { get; set; }
public string Surname { get; set; }
public Address Address { get; set; }
public List<Preference> Preferences { get; set; }
}
public class Address
{
public string ResidentialAddress{ get; set; }
public string PostalAddress{ get; set; }
}
public class Preference
{
public string Name{ get; set; }
public string Description{ get; set; }
}
DTO - Data Transfer Object
DTO is short for Data Transfer Object and is one of the most common objects for transferring data between layers in your applications (infrastructure, core, and host - specific for onion architecture).
If you have ever worked with DTOs before you probably know they usually are immutable and they got no logic. DTOs are only used as data-only objects for transferring data around in the application. Often you will see them used with AutoMapper in .NET where we map data from repositories and services to controllers.
If you take a look at an entity above you can see they reflect a model for our domain or business logic in the application. A DTO on the other hand will not be responsible for such things, they will only reflect what the consumer requesting the data needs.
DTOs are often created for a very specific purpose where we return or request data from a service. A DTO can get enriched with data from multiple sources in order to fulfill the consumer's needs.
VO - Value Object
A VO (Value Object) is an immutable object we can use to hold data. Okay... So how is a Value Object different from a Data Transfer Object? A VO is capable of representing an Entity and as you might remember, an entity got responsibility and its own behavior.
Christian - Why don't we just use Value Objects, if they are the same as an entity and a DTO? Well, they are not. 😅 A Value Object is not allowed to be used to persist data or connect to databases or any other kind of external/internal storage mechanism for the application.
In other words, a Value Object is only responsible for transferring data and has to make sure the data is in a valid state to be used with an Entity. It's immutable and provides only the information that the consuming service is requesting.
Responsibility Overview
I have summed up the above descriptions of the classes into a table for you to easily see the differences between their responsibilities.
Database | Responsibilities | Immutable | |
---|---|---|---|
POCO | ❌ | ✅ | ❌ |
Entity | ✅ | ✅ | ❌ |
DTO | ❌ | ❌ | ✅ |
VO | ❌ | ❌ | ✅ |
Summary
You made it to the end! Awesome, thank you for reading this far. You should now have a better understanding of each type and its responsibilities. As I mentioned at the very beginning of the article you will see these responsibilities change from programming language to programming language. Often they will stay much the same for the popular programming languages.
I hope you learned something from this short article about the type of classes: DTO VO, Entity, and POCO. You will hear them often referenced by other developers in documentation or explanations of software. Especially on Stack Overflow are other developers using abbreviations a lot!
If you got any questions about the content of this article, please let me know in the comments below. Until next time - Happy coding! ✌️